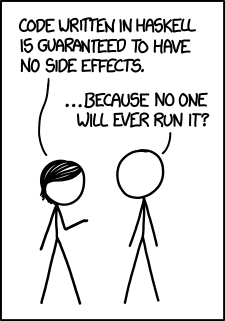
A lesson from open source software
I've always tried to contribute to open source software whenever I've seen the opportunity, but becoming a maintainer of an open source library gave me some completely new perspectives.
I've used Emacs for almost a decade, and I used Vim before that. Maybe the fact that vi key bindings are just another package in Emacs, called Evil, was one of the reasons I switched to Emacs. I like modal editing, but I don't use Evil. Instead, I use God mode with Emacs key bindings.
At some point, I discovered Doom Emacs, which packed a lot of functionality that interested me compared to other Emacs distributions.
Continue reading →Can typeclasses solve the expression problem?
Typeclasses in Haskell roughly correspond to interfaces in object-oriented languages and can be used to solve the Expression Problem. More specifically, since Haskell takes parametric polymorphism quite seriously, typeclasses are more akin to generic interfaces, but also allow ad-hoc polymorphism.
Let's first define an Expr
typeclass with const
and add
as functions. These functions represent types or variants in the Expression Problem.
class Expr t where
const :: Double -> t
add :: t -> t -> t
Continue reading →
Can object algebras solve the expression problem?
Another way to solve the expression problem in C# and other object-oriented languages is by using object algebras[1]. Object algebras define a parameterised interface, and a factory that implements that interface.
First, let's define the interface of the Eval
operation.
public interface IEval
{
double Eval();
}
Continue reading →
Can extension methods solve the expression problem?
Let's explore how extension methods in C# can solve the expression problem. For an introduction to the expression problem, refer to my first post in this series. Extension methods allow operations to be defined over a type without modifying the type's original definition.
First, we define IExpr
as a marker interface and implement it in the Const
and Add
types. The implementations of Const
and Add
here are identical to those in the previous post.
public interface IExpr { }
public class Const : IExpr
{
public double Value { get; }
public Const(double value) =>
Value = value;
}
public class Add : IExpr
{
public IExpr Left { get; }
public IExpr Right { get; }
public Add(IExpr left, IExpr right) =>
(Left, Right) = (left, right);
}
Continue reading →